mirror of
https://github.com/bitcoin/bitcoin.git
synced 2025-01-28 03:57:31 -03:00
af112ab628
qt: Rename SetPrune() to InitializePruneSetting() (Hennadii Stepanov)b0bfbe5028
refactor: Drop `bool force' parameter (Hennadii Stepanov)68c9bbe9bc
qt: Force set nPruneSize in QSettings after intro (Hennadii Stepanov)a82bd8fa57
util: Replace magics with DEFAULT_PRUNE_TARGET_GB (Hennadii Stepanov) Pull request description: On master (5622d8f315
), having `QSettings` set already ``` $ grep nPruneSize ~/.config/Bitcoin/Bitcoin-Qt-testnet.conf nPruneSize=6 ``` enabling prune option in the intro dialog ``` $ ./src/qt/bitcoin-qt -choosedatadir -testnet ``` 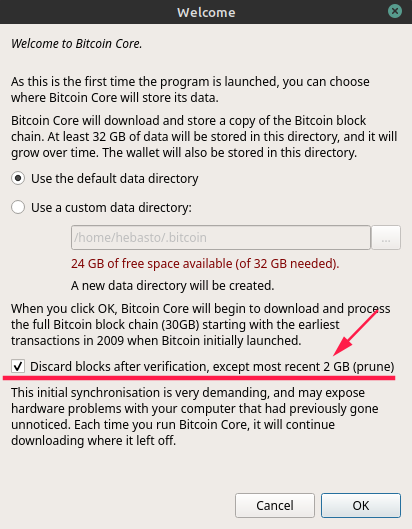 has no effect: ``` $ grep Prune ~/.bitcoin/testnet3/debug.log 2019-12-08T10:04:41Z Prune configured to target 5722 MiB on disk for block and undo files. ``` --- With this PR: ``` $ grep Prune ~/.bitcoin/testnet3/debug.log 2019-12-08T10:20:35Z Prune configured to target 1907 MiB on disk for block and undo files. ``` This PR has been split of #17453 (the first two commits) as it fixes an orthogonal bug. Refs: - https://github.com/bitcoin/bitcoin/pull/17453#discussion_r345424240 - https://github.com/bitcoin/bitcoin/pull/17453#discussion_r350960201 ACKs for top commit: Sjors: Code review re-ACKaf112ab628
ryanofsky: Code review ACKaf112ab628
. Just suggested changes since last review (thanks!) promag: Tested ACKaf112ab628
. Latest suggestions and changes look good to me. Tree-SHA512: 8ddad34b30dcc2cdcad6678ba8a0b36fa176e4e3465862ef6eee9be0f98d8146705138c9c7995dd8c0990af41078ca743fef1a90ed9240081f052f32ddec72b9
125 lines
3.2 KiB
C++
125 lines
3.2 KiB
C++
// Copyright (c) 2011-2019 The Bitcoin Core developers
|
|
// Distributed under the MIT software license, see the accompanying
|
|
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
|
|
|
|
#ifndef BITCOIN_QT_BITCOIN_H
|
|
#define BITCOIN_QT_BITCOIN_H
|
|
|
|
#if defined(HAVE_CONFIG_H)
|
|
#include <config/bitcoin-config.h>
|
|
#endif
|
|
|
|
#include <QApplication>
|
|
#include <memory>
|
|
|
|
class BitcoinGUI;
|
|
class ClientModel;
|
|
class NetworkStyle;
|
|
class OptionsModel;
|
|
class PaymentServer;
|
|
class PlatformStyle;
|
|
class WalletController;
|
|
class WalletModel;
|
|
|
|
namespace interfaces {
|
|
class Handler;
|
|
class Node;
|
|
} // namespace interfaces
|
|
|
|
/** Class encapsulating Bitcoin Core startup and shutdown.
|
|
* Allows running startup and shutdown in a different thread from the UI thread.
|
|
*/
|
|
class BitcoinCore: public QObject
|
|
{
|
|
Q_OBJECT
|
|
public:
|
|
explicit BitcoinCore(interfaces::Node& node);
|
|
|
|
public Q_SLOTS:
|
|
void initialize();
|
|
void shutdown();
|
|
|
|
Q_SIGNALS:
|
|
void initializeResult(bool success);
|
|
void shutdownResult();
|
|
void runawayException(const QString &message);
|
|
|
|
private:
|
|
/// Pass fatal exception message to UI thread
|
|
void handleRunawayException(const std::exception *e);
|
|
|
|
interfaces::Node& m_node;
|
|
};
|
|
|
|
/** Main Bitcoin application object */
|
|
class BitcoinApplication: public QApplication
|
|
{
|
|
Q_OBJECT
|
|
public:
|
|
explicit BitcoinApplication(interfaces::Node& node);
|
|
~BitcoinApplication();
|
|
|
|
#ifdef ENABLE_WALLET
|
|
/// Create payment server
|
|
void createPaymentServer();
|
|
#endif
|
|
/// parameter interaction/setup based on rules
|
|
void parameterSetup();
|
|
/// Create options model
|
|
void createOptionsModel(bool resetSettings);
|
|
/// Initialize prune setting
|
|
void InitializePruneSetting(bool prune);
|
|
/// Create main window
|
|
void createWindow(const NetworkStyle *networkStyle);
|
|
/// Create splash screen
|
|
void createSplashScreen(const NetworkStyle *networkStyle);
|
|
/// Basic initialization, before starting initialization/shutdown thread. Return true on success.
|
|
bool baseInitialize();
|
|
|
|
/// Request core initialization
|
|
void requestInitialize();
|
|
/// Request core shutdown
|
|
void requestShutdown();
|
|
|
|
/// Get process return value
|
|
int getReturnValue() const { return returnValue; }
|
|
|
|
/// Get window identifier of QMainWindow (BitcoinGUI)
|
|
WId getMainWinId() const;
|
|
|
|
/// Setup platform style
|
|
void setupPlatformStyle();
|
|
|
|
public Q_SLOTS:
|
|
void initializeResult(bool success);
|
|
void shutdownResult();
|
|
/// Handle runaway exceptions. Shows a message box with the problem and quits the program.
|
|
void handleRunawayException(const QString &message);
|
|
|
|
Q_SIGNALS:
|
|
void requestedInitialize();
|
|
void requestedShutdown();
|
|
void splashFinished();
|
|
void windowShown(BitcoinGUI* window);
|
|
|
|
private:
|
|
QThread *coreThread;
|
|
interfaces::Node& m_node;
|
|
OptionsModel *optionsModel;
|
|
ClientModel *clientModel;
|
|
BitcoinGUI *window;
|
|
QTimer *pollShutdownTimer;
|
|
#ifdef ENABLE_WALLET
|
|
PaymentServer* paymentServer{nullptr};
|
|
WalletController* m_wallet_controller{nullptr};
|
|
#endif
|
|
int returnValue;
|
|
const PlatformStyle *platformStyle;
|
|
std::unique_ptr<QWidget> shutdownWindow;
|
|
|
|
void startThread();
|
|
};
|
|
|
|
int GuiMain(int argc, char* argv[]);
|
|
|
|
#endif // BITCOIN_QT_BITCOIN_H
|